Hadoop入门教程(六):Hadoop API 使用编程的方式操作 HDFS
2021年03月15日 11:05:18 · 本文共 4,201 字阅读时间约 15分钟 · 2,940 次浏览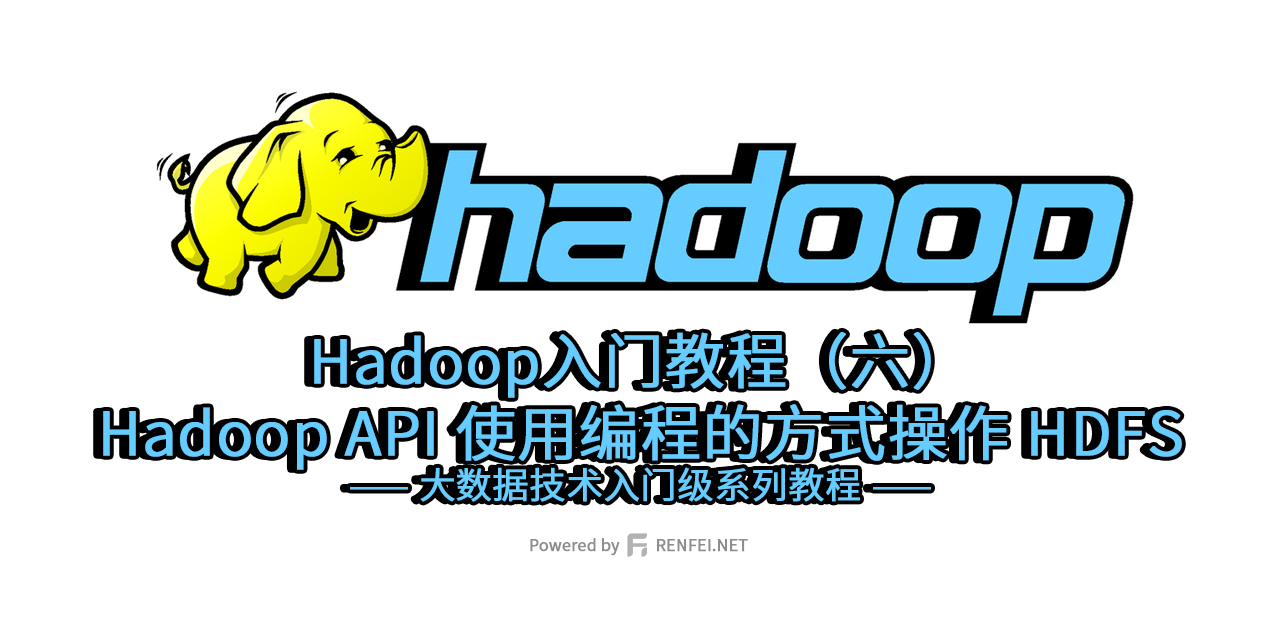
教程索引目录请访问:《大数据技术入门级系列教程》
上一篇讲了使用 Shell 命令操作 HDFS,但实际中我们肯定不可能一直手动操作,还是需要通过编程实现自动化的,所以本文将带你熟悉一下使用 Java 编程控制 HDFS 中的文件。
先决条件
本章内容默认读者已经具备 Java 编程能力,其中包括 JavaSE 基础知识、Maven 构建知识,如果您还不会使用 Java/Maven 构建项目,请先学习相关知识。并且已经阅读过前面的章节搭建起了 Hadoop 平台,如果您还没有搭建起 Hadoop 平台,可能无法操作本章的内容。本章的完整代码分享在:https://github.com/renfei/demo/tree/master/hadoop/hadoop_api
Maven项目创建
我们需要先创建一个 Maven 项目,并引用相关的依赖,Maven 是什么在这里不讨论了,主要讨论 Hadoop 的 HDFS,请自行学习 Maven,这里我贴一下 Maven 的 pom.xml 中的依赖:
<properties>
<hadoop.version>2.10.1</hadoop.version>
<log4j.version>2.14.0</log4j.version>
</properties>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>RELEASE</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-common</artifactId>
<version>${hadoop.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-client</artifactId>
<version>${hadoop.version}</version>
</dependency>
<dependency>
<groupId>org.apache.hadoop</groupId>
<artifactId>hadoop-hdfs</artifactId>
<version>${hadoop.version}</version>
</dependency>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>${log4j.version}</version>
</dependency>
</dependencies>
HDFS的API操作
HDFS创建文件夹
public void mkdirs() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
fileSystem.mkdirs(new Path("/demo"));
fileSystem.close();
}
HDFS文件上传
public void upload() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
fileSystem.copyFromLocalFile(new Path("/Users/renfei/Downloads/demo.txt"), new Path("/demo/demo.txt"));
fileSystem.close();
}
HDFS文件下载
public void get() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
fileSystem.copyToLocalFile(new Path("/demo/demo.txt"), new Path("/Users/renfei/Downloads/demo2.txt"));
fileSystem.close();
}
HDFS文件重命名
public void rename() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
fileSystem.rename(new Path("/demo/demo.txt"), new Path("/demo/demo2.txt"));
fileSystem.close();
}
HDFS文件详情获取
public void listFiles() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
RemoteIterator<LocatedFileStatus> listFiles = fileSystem.listFiles(new Path("/demo/"), true);
while (listFiles.hasNext()) {
LocatedFileStatus status = listFiles.next();
// 文件名称
System.out.println(status.getPath().getName());
// 长度
System.out.println(status.getLen());
// 权限
System.out.println(status.getPermission());
// 分组
System.out.println(status.getGroup());
// 获取存储的块信息
BlockLocation[] blockLocations = status.getBlockLocations();
for (BlockLocation blockLocation : blockLocations) {
// 获取块存储的主机节点
String[] hosts = blockLocation.getHosts();
for (String host : hosts) {
System.out.println(host);
}
}
System.out.println("---------------------");
}
fileSystem.close();
}
HDFS文件删除
public void delete() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
// 此处第二参数 true 是指递归删除
if (fileSystem.delete(new Path("/demo"), true)) {
System.out.println("删除成功");
} else {
System.out.println("删除失败");
}
fileSystem.close();
}
HDFS文件流式上传
public void upload() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
// 创建一个文件输入流
FileInputStream fileInputStream = new FileInputStream(new File("/Users/renfei/Downloads/demo.txt"));
// 获取输出流
FSDataOutputStream fsDataOutputStream = fileSystem.create(new Path("/demo/demo.txt"));
// 流拷贝
IOUtils.copyBytes(fileInputStream, fsDataOutputStream, configuration);
// 关闭资源
IOUtils.closeStream(fsDataOutputStream);
IOUtils.closeStream(fileInputStream);
fileSystem.close();
}
HDFS文件流式下载
public void get() throws IOException, InterruptedException {
FileSystem fileSystem = FileSystem.get(uri, configuration, user);
// 获取输入流
FSDataInputStream fsDataOutputStream = fileSystem.open(new Path("/demo/demo.txt"));
// 获取输出流
FileOutputStream fileOutputStream = new FileOutputStream(new File("/Users/renfei/Downloads/demo2.txt"));
// 流拷贝
IOUtils.copyBytes(fsDataOutputStream, fileOutputStream, configuration);
// 关闭资源
IOUtils.closeStream(fsDataOutputStream);
IOUtils.closeStream(fileOutputStream);
fileSystem.close();
}
商业用途请联系作者获得授权。
版权声明:本文为博主「任霏」原创文章,遵循 CC BY-NC-SA 4.0 版权协议,转载请附上原文出处链接及本声明。
原文链接:https://www.renfei.net/posts/1003464
版权声明:本文为博主「任霏」原创文章,遵循 CC BY-NC-SA 4.0 版权协议,转载请附上原文出处链接及本声明。
原文链接:https://www.renfei.net/posts/1003464
相关推荐
猜你还喜欢这些内容,不妨试试阅读一下
评论与留言
以下内容均由网友提交发布,版权与真实性无法查证,请自行辨别。
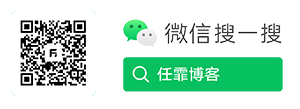
热评文章
- 前后端分离项目接口数据加密的秘钥交换逻辑(RSA、AES)
- OmniGraffle 激活/破解 密钥/密匙/Key/License
- CleanMyMac X 破解版 [TNT] 4.6.0
- OmniPlan 激活/破解 密钥/密匙/Key/License
- Parallels Desktop For Mac 16.0.1.48911 破解版 [TNT]
- Redis 未授权访问漏洞分析 cleanfda 脚本复现漏洞挖矿
- Parallels Desktop For Mac 15.1.4.47270 破解版 [TNT]
- 人大金仓 KingbaseES V8 R3 安装包、驱动包和 License 下载地址
- Sound Control 破解版 2.4.2
- 向谷歌搜索引擎主动推送网页的教程 Google Indexing API 接口实现
热文排行
- 博客完全迁移上阿里云,我所使用的阿里云架构
- 微软确认Windows 10存在bug 部分电脑升级后被冻结
- 大佬们在说的AQS,到底啥是个AQS(AbstractQueuedSynchronizer)同步队列
- 比特币(BTC)钱包客户端区块链数据同步慢,区块链数据离线下载
- Java中说的CAS(compare and swap)是个啥
- 小心免费主题!那些WordPress主题后门,一招拥有管理员权限
- 强烈谴责[wamae.win]恶意反向代理我站并篡改我站网页
- 讨论下Java中的volatile和JMM(Java Memory Model)Java内存模型
- 新版个人网站 NEILREN4J 上线并开源程序源码
- 我站近期遭受到恶意不友好访问攻击公告